Level Up Your Data Analysis: Breaking 10 Old Pandas Habits
Written on
Chapter 1: Introduction
For data analysts, becoming proficient in Pandas is vital for effective data manipulation. However, certain outdated practices can impede your progress. Here, I will outline ten old habits I have abandoned to elevate my data analysis skills.
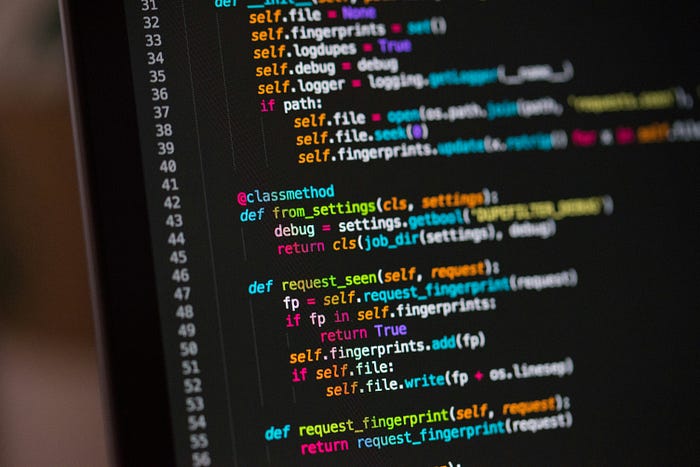
Section 1.1: Habit 1 - Overusing .iterrows()
Relying heavily on .iterrows() can slow down your processing speed. Instead, consider using vectorized operations for enhanced performance.
# Old Approach
for index, row in df.iterrows():
# process row
# Improved Method
Section 1.2: Habit 2 - Excessive Chaining of Operations
Chaining too many operations can lead to convoluted code. Simplifying your code into smaller, more manageable sections enhances readability.
# Old Approach
result = df[df['column1'] > 0].groupby('column2').mean().reset_index()
# Improved Method
filtered_df = df[df['column1'] > 0]
grouped_df = filtered_df.groupby('column2').mean()
result = grouped_df.reset_index()
Section 1.3: Habit 3 - Unnecessary Use of apply()
The apply() function can be inefficient. Opt for vectorized operations wherever feasible.
# Old Approach
df['new_column'] = df['old_column'].apply(lambda x: my_function(x))
# Improved Method
df['new_column'] = my_function(df['old_column'])
Section 1.4: Habit 4 - Ignoring .loc and .iloc
Directly assigning values without using .loc or .iloc can lead to warnings and unintended behavior.
# Old Approach
df[df['column'] > 0]['new_column'] = value
# Improved Method
df.loc[df['column'] > 0, 'new_column'] = value
Section 1.5: Habit 5 - Mishandling Missing Values
Failing to address missing values can distort your analysis. Utilize methods like fillna() or dropna() for better handling.
# Old Approach
mean_value = df['column'].mean()
# Improved Method
mean_value = df['column'].fillna(0).mean()
Section 1.6: Habit 6 - Inefficient Row Looping
Iterating through DataFrame rows is not an optimal approach. Seek out vectorized alternatives.
# Old Approach
for i in range(len(df)):
# process row
# Improved Method
for index, row in df.iterrows():
# process row
Chapter 2: More Outdated Practices
Section 2.1: Habit 7 - Misusing .at and .iat
Using .loc or .iloc for scalar access is less efficient than using .at or .iat.
# Old Approach
value = df.loc[0, 'column']
# Improved Method
value = df.at[0, 'column']
Section 2.2: Habit 8 - Confusion with inplace Parameter
Utilizing inplace=True can often lead to misunderstandings. It is usually clearer to use assignment instead.
# Old Approach
df.dropna(inplace=True)
# Improved Method
df = df.dropna()
Section 2.3: Habit 9 - Inefficient Aggregation with groupby()
Using groupby().apply() for straightforward aggregations is less efficient than built-in functions like mean() or sum().
# Old Approach
result = df.groupby('column').apply(lambda x: x['value'].sum())
# Improved Method
result = df.groupby('column')['value'].sum()
Section 2.4: Habit 10 - Overlooking Pandas Documentation
The Pandas documentation is a treasure trove of valuable functions and methods. Regularly exploring it can lead to discovering more efficient techniques.
# Old Approach
struggling with a problem
# Improved Method
consulting Pandas documentation for solutions
By eliminating these outdated habits, I have greatly enhanced my data analysis process, making it both more efficient and reliable. Embrace these changes to advance your own data analysis capabilities!
Learn how to solve 100 Python Pandas challenges, ranging from easy to very difficult, in this engaging video.
In just 10 minutes, gain insights into Python data analysis with Pandas through this quick tutorial by Udemy instructor Frank Kane.