Mastering Ref Forwarding in React with TypeScript
Written on
Understanding References in React
In React, references play a crucial role. They enable you to maintain a reference to a specific node or element, allowing access to its properties. For example, consider the following code snippet:
const reference = useRef<HTMLDivElement>(null);
return (
<>
<div ref={reference}>
...</div>
<div style={{ height: reference?.current?.clientHeight + 'px' }}>
...</div>
</>
);
Here, we have two simple div elements. The first div is assigned a reference named reference. Consequently, we can extract data from it and use that information to style the second div accordingly. This is a straightforward and common task.
But how can we pass these references to another component?
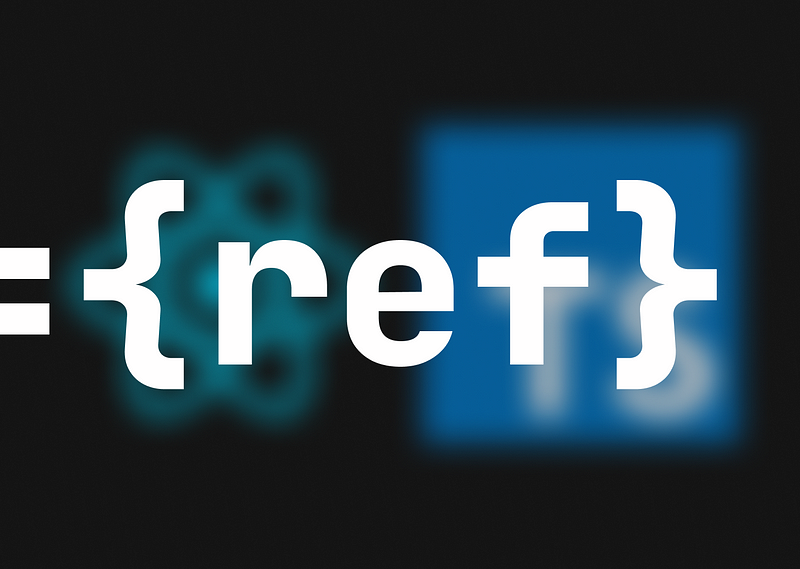
While React allows the passing of props, you might think that sending the reference through a prop named ref to another component would be a feasible solution. However, that’s not the case. Here’s what it would look like:
const reference = useRef<HTMLDivElement>(null);
const SomeComponent = (props: { ref: any }) => {
return (
<div ref={props.ref}>
...</div>
);
};
return (
<>
...
<SomeComponent ref={reference} />
...
</>
);
If you were to log props.ref within SomeComponent, it would return undefined.
The solution lies in using the React.forwardRef function.
What is React.forwardRef? This function facilitates the passing of references from parent to child components. Here's how you can implement it:
const ChildComponent = React.forwardRef(
(props: { someProp: any }, ref: React.Ref<HTMLDivElement>) => {
return (
<div ref={ref}>
...</div>
);
}
);
const ParentComponent = () => {
const reference = useRef<HTMLDivElement>(null);
return (
<>
...
<ChildComponent ref={reference} someProp={...} />
<div style={{ height: reference?.current?.innerHeight }} />
...
</>
);
};
In this scenario, ChildComponent successfully recognizes the reference, enabling the ParentComponent to utilize it for styling, scrolling actions, and more.
Learn about ref forwarding in React from this informative video titled "A Trick Every React Developer Should Know: Ref Forwarding."
Explore how to effectively pass the forwardRef hook between multiple components in React through this insightful video.
In conclusion, I hope this article has been beneficial as you explore new learning materials. Thank you for taking the time to read it!
Feel free to subscribe to my email list or follow me on Twitter for more engaging content in the future!