Innovative Insights: Comparing Python, Julia, and Rust
Written on
Chapter 1: Overview of Modern Programming Languages
Python, Julia, and Rust are three contemporary programming languages that present both commonalities and notable distinctions. This section offers a detailed analysis of each language, complete with examples that showcase their principal attributes.
Section 1.1: Syntax Comparison
Python is celebrated for its straightforward and readable syntax, often likened to "executable pseudocode." It utilizes indentation to define code blocks, eliminating the need for curly braces or semicolons. Below is an example of a basic function written in Python:
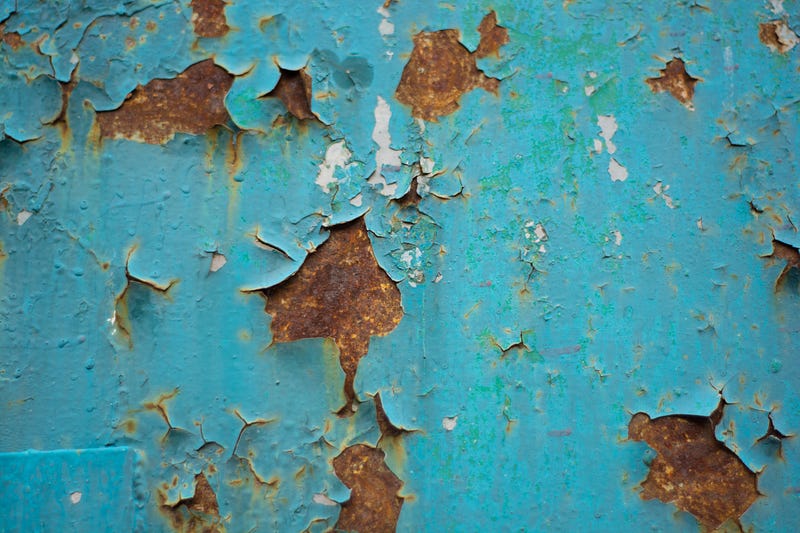
def greet(name):
print("Hello, " + name + "!")
greet("Alice")
In contrast, Julia adopts a syntax reminiscent of Python but incorporates elements from C and Fortran. For instance, it permits the use of semicolons to separate statements and curly braces for code blocks. Here’s a simple function in Julia:
function greet(name)
println("Hello, $name!")
end
greet("Alice")
Rust, on the other hand, has a syntax akin to C++, enriched with features that bolster its robust static typing and memory safety. It employs curly braces for code blocks and semicolons to separate statements, often resulting in more verbose code due to explicit type annotations. Here’s an example in Rust:
fn greet(name: &str) {
println!("Hello, {}!", name);
}
greet("Alice");
Section 1.2: Performance and Execution Speed
Julia stands out for its speed, leveraging just-in-time (JIT) compilation for optimal performance. This allows Julia code to be compiled to native machine code during execution, potentially surpassing the speed of Python and other interpreted languages. Furthermore, Julia includes optimized routines tailored for numerical and scientific computations, enhancing its performance.
Rust is also recognized for its speed, particularly in CPU-intensive tasks. Being a compiled language, Rust translates code to machine code prior to execution, which generally makes it faster than interpreted languages like Python. It features zero-cost abstractions and minimal runtime overhead, contributing to its performance.
Conversely, Python is typically slower than both Julia and Rust. However, its performance can be boosted with libraries like NumPy and Cython. As an interpreted language, Python executes code directly through an interpreter, which can lead to slower performance in CPU-heavy tasks.
Chapter 2: Memory Management Techniques
Rust places a significant emphasis on memory safety through features such as borrowing and ownership, which help to avoid common memory issues like null or dangling pointer references. Its borrowing system allows multiple references to the same data while restricting modifications through all but one reference, facilitating safe concurrent code development.
Julia features an efficient garbage collector that automatically manages memory, similar to Python, allowing developers to concentrate on coding without worrying about memory management.
Python also employs a garbage collector for automatic memory management. While this can be less efficient than Rust’s approach, it simplifies memory management for developers. However, Python’s garbage collector may occasionally cause delays during critical code execution.
The video titled "Stephan Sahm: Accelerate Python with Julia" explores how Julia can enhance Python's performance, illustrating the integration of these two languages.
Chapter 3: Concurrency Capabilities
Rust excels in concurrent programming, providing features like threads and message passing to enable parallel task execution. Its ownership and borrowing system simplifies the management of shared resources, making it easier to write safe concurrent applications. Below is a snippet of a concurrent Rust program that computes the sum of a large array in parallel:
use std::sync::{Arc, Mutex};
use std::thread;
fn main() {
let data = Arc::new(Mutex::new(vec![1, 2, 3, 4, 5]));
let mut handles = vec![];
for i in 0..5 {
let data = data.clone();
handles.push(thread::spawn(move || {
let mut data = data.lock().unwrap();
data[i] += 1;
}));
}
for handle in handles {
handle.join().unwrap();}
let data = data.lock().unwrap();
let sum: i32 = data.iter().sum();
println!("Sum: {}", sum);
}
Julia also supports concurrency effectively with features like asynchronous tasks and distributed computing. Its task system allows developers to designate code segments for parallel execution, managing scheduling and execution effortlessly. Here’s an example of a simple concurrent program in Julia:
using Distributed
@everywhere function addone(x)
return x + 1
end
function main()
data = [1, 2, 3, 4, 5]
data = @parallel (addone) for x in data
sum = sum(data)
println("Sum: $sum")
end
main()
Python offers concurrency options, but its performance can be hindered by the global interpreter lock (GIL). The threading module allows for thread creation and management, yet only one thread can execute Python bytecode simultaneously. Alternatively, the multiprocessing module enables process management, which can be more effective for concurrent tasks but comes with added overhead. Below is an example of a concurrent Python program using the multiprocessing module:
from multiprocessing import Process, Manager
def addone(data, i):
data[i] += 1
def main():
manager = Manager()
data = manager.list([1, 2, 3, 4, 5])
processes = []
for i in range(5):
p = Process(target=addone, args=(data, i))
processes.append(p)
p.start()
for p in processes:
p.join()
sum = sum(data)
print("Sum:", sum)
main()
The video "Python vs Julia" delves into the differences between these two languages, exploring their strengths and weaknesses in various programming contexts.
Chapter 4: Practical Applications
Rust is frequently utilized in system-level programming, including operating systems, device drivers, and low-level libraries. Its robust static typing and memory safety make it ideal for performance-sensitive tasks, such as systems programming and high-concurrency applications.
Julia is preferred for numerical and scientific computing, along with machine learning and data analysis. Its high performance in managing large datasets makes it suitable for scientific simulations and data-intensive tasks, bolstered by numerous libraries for machine learning and data visualization.
Python is a versatile language widely used in web development, scientific computing, data analysis, and machine learning. Its simplicity and extensive library support make it an excellent choice for various projects, particularly those involving data processing or rapid prototyping.
In conclusion, Python, Julia, and Rust are all potent programming languages, each with distinct advantages and limitations. While Julia and Rust are tailored for high-performance tasks, Python's versatility and ease of learning position it as a favorable option for many types of projects. The ideal language choice ultimately hinges on the specific requirements and objectives of the undertaking.