Setting Up HTTPS in Your Spring Boot Application Step-by-Step
Written on
Chapter 1: Introduction to HTTPS in Spring Boot
In this tutorial, we will explore the process of enabling HTTPS for your Spring Boot applications using self-signed certificates. Up to now, our Spring Boot applications have been accessible via HTTP. We have covered HTTP in previous posts, and this article will focus on transforming an HTTP endpoint into an HTTPS one.
Before diving into the implementation, let's clarify a few essential concepts.
Section 1.1: Understanding SSL
SSL, or Secure Sockets Layer, is a protocol designed to secure data transmitted over the internet between two devices. By encrypting data, SSL ensures that it remains protected from unauthorized access. Although SSL has been succeeded by TLS (Transport Layer Security), the term SSL is still commonly used, and the certificates are often referred to as SSL/TLS certificates.
Subsection 1.1.1: The Role of SSL Certificates
An SSL certificate serves to authenticate a server, allowing clients to verify the server's identity. Each certificate contains a pair of cryptographic keys: a public key, which is shared with clients, and a private key, which is securely held on the server. Clients use the public key to encrypt data, while the server utilizes the private key to decrypt it. While various authorities issue these certificates, we will create a self-signed certificate for this tutorial.
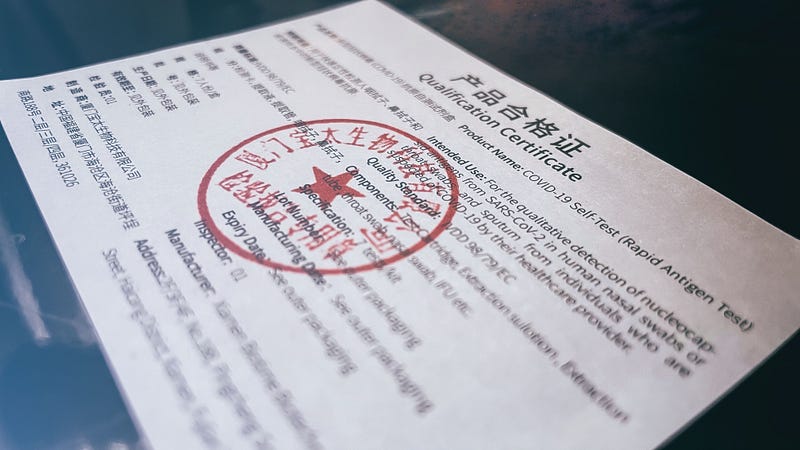
Section 1.2: KeyStore in Java
In Java, a KeyStore is a repository for storing private keys and public key certificates. The default format for KeyStore since Java 9 is PKCS12, which we will use in this guide.
Chapter 2: Generating Self-Signed Certificates
We can utilize tools like OpenSSL or keytool to create self-signed certificates. Keep in mind that browsers may display warnings when connecting to servers with self-signed certificates, and tools like Postman may not accept them.
To generate a self-signed certificate, use the following command in your terminal:
keytool -genkeypair -alias samplekey -keyalg RSA -keysize 2048 -storetype PKCS12 -keystore samplekey.p12 -validity 3650
After executing this command, you will be prompted to answer several questions. Remember the password, as it is necessary for your properties file.
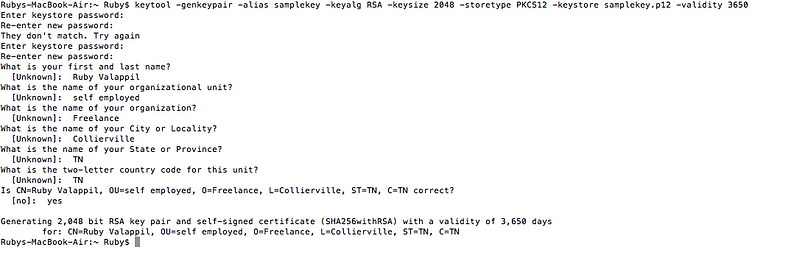
Next, create a Spring Boot project and implement a simple GET API. Place your certificate in the resources folder under a new subdirectory named "keystore" (i.e., src/main/resources/keystore). In your application.properties file, add the following lines:
server.ssl.key-store-type=PKCS12
server.ssl.key-store=classpath:keystore/samplekey.p12
server.ssl.key-store-password=<Your Password>
server.ssl.key-alias=samplekey
server.ssl.enabled=true
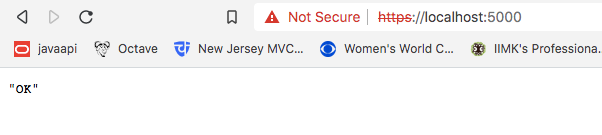
If you attempt to access the API over HTTP, you will receive a 400 error.
Chapter 3: Testing with Postman
Postman allows the inclusion of client certificates, but it may struggle with self-signed certificates created with the PKCS12 format. I attempted to convert the certificate to PEM format and use it as a CA certificate in Postman, but it was unsuccessful.
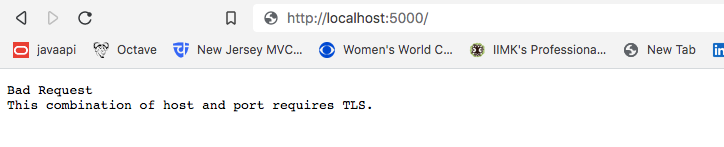
To bypass SSL verification in Postman, simply disable the SSL certificate validation option.
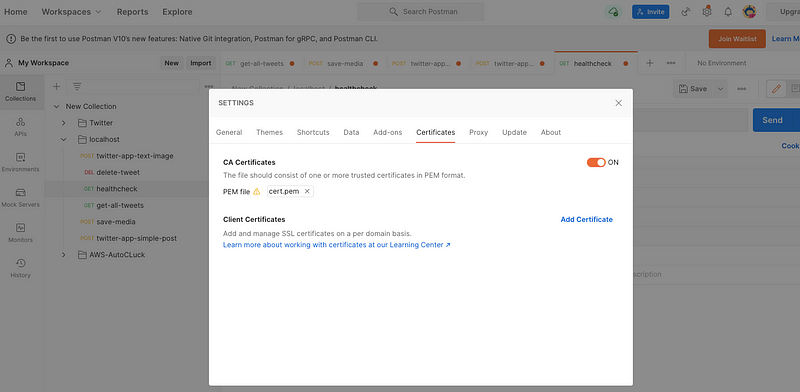
Once SSL verification is disabled, you should receive the expected response when you access the GET API.
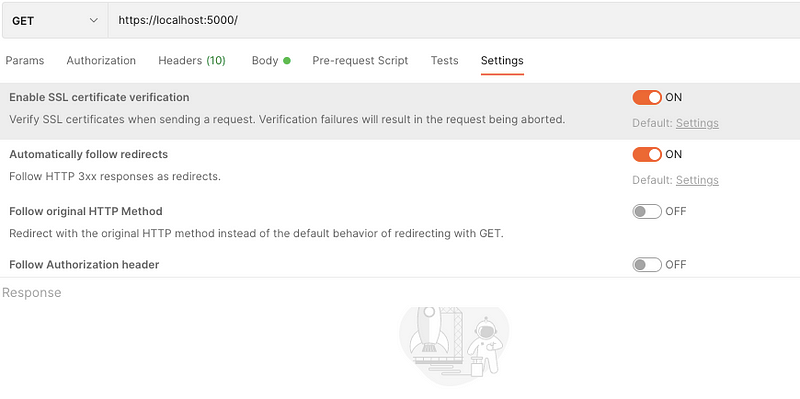
To further enhance your knowledge and stay updated with the latest technology trends, consider subscribing to my Weekly Free Newsletter for Techies.
Chapter 4: Additional Resources
The first video, "Enable HTTPS/SSL in Spring Boot," offers a comprehensive overview of the steps involved in securing your Spring Boot application with HTTPS.
In the second video, "Spring Boot with HTTPS Example | Tech Primers," you will find practical examples and further explanations on implementing HTTPS in Spring Boot applications.